In this tutorial, you will learn how to play mp3 on your FeatherWing board using CircuitPython.
Parts you need in this tutorial:
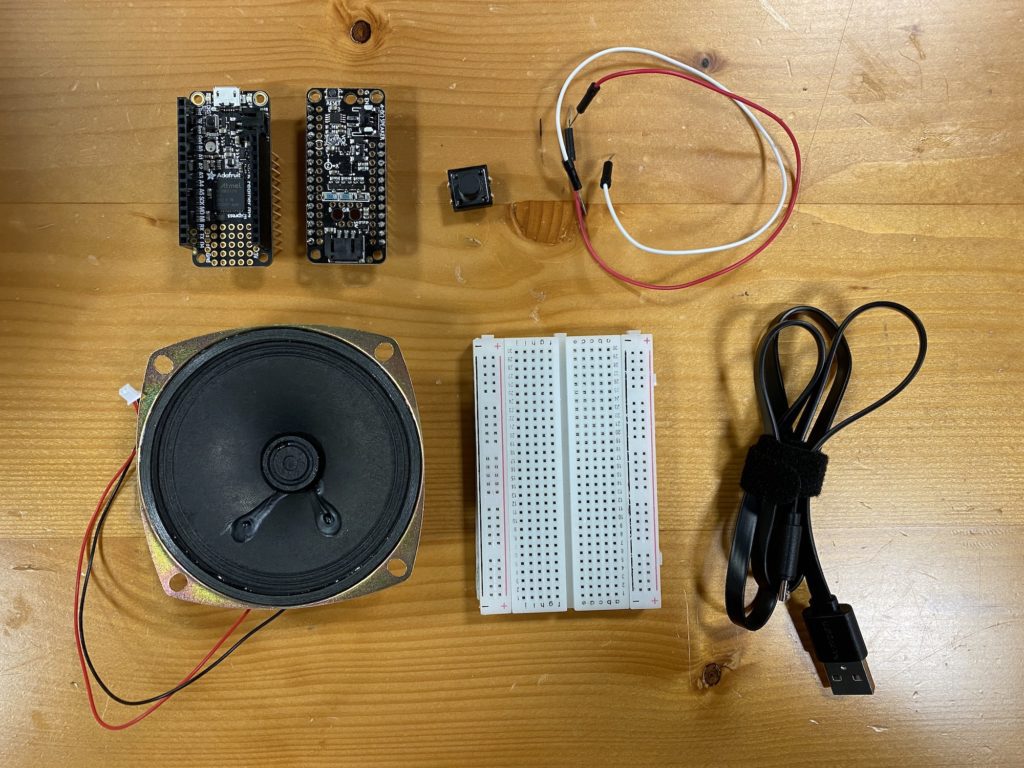
- Adafruit Feather M4 Express
- Adafruit Prop-Maker FeatherWing
- Breadboard
- Push-button
- 4Ohm 3W Speaker (with Pico Blade 2-pin connector)
- Male-Male jumper wire
- USB – Micro USB connector cable
Preview:
Step 0: Pre-soldering
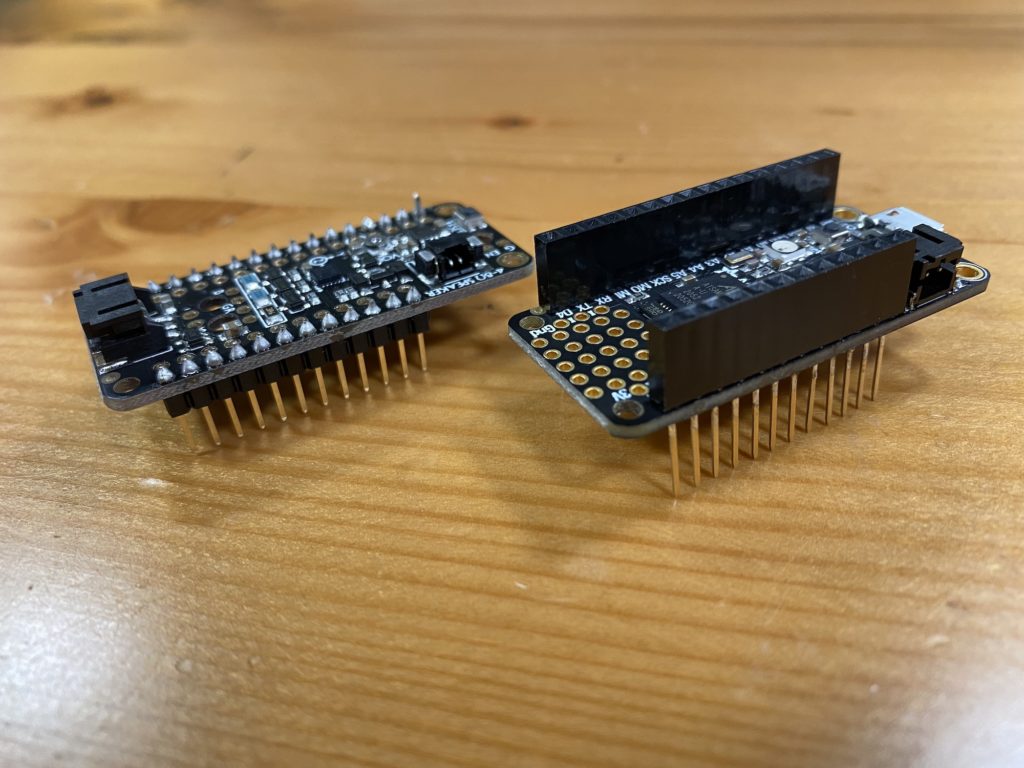
Add header pins to your M4 Express and Prop-Maker boards. Usually the header pins come with the board in the same packet.
The M4 Express should be soldered with Female-Male feather stacking headers. And the Prop-Maker should be soldered with Male-Male headers.
Check this guide to see how to solder the headers.
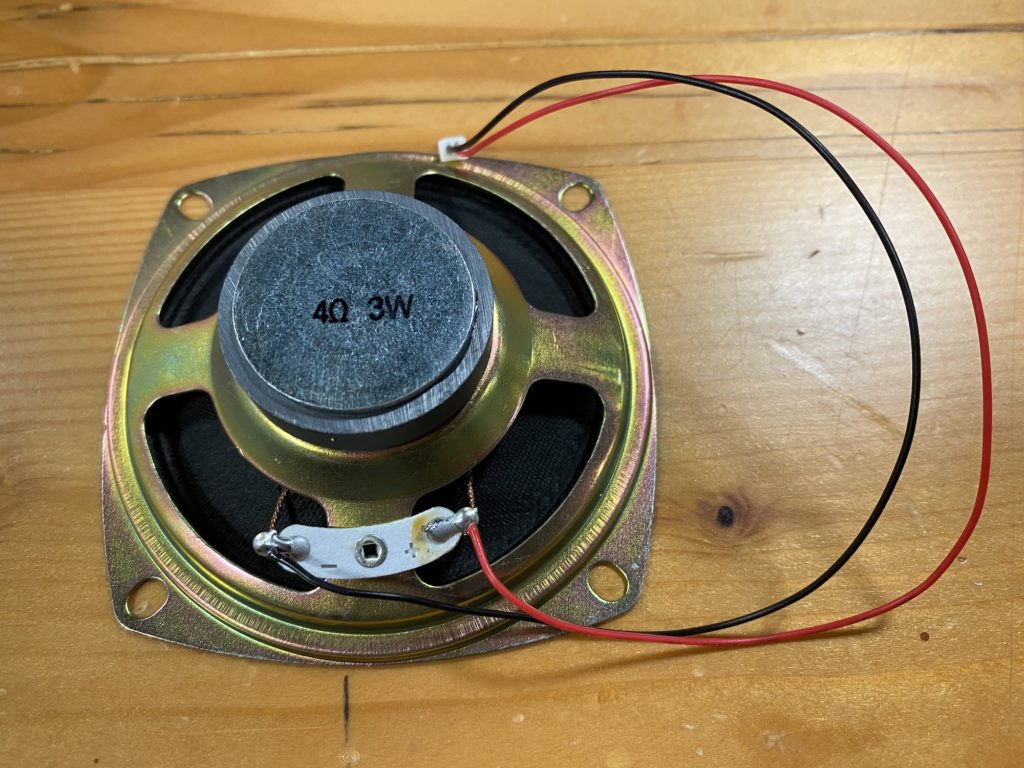
Add Pico Blade 2-pin connector to the speaker.
Step 1: Setup Circuit Python on M4 Express
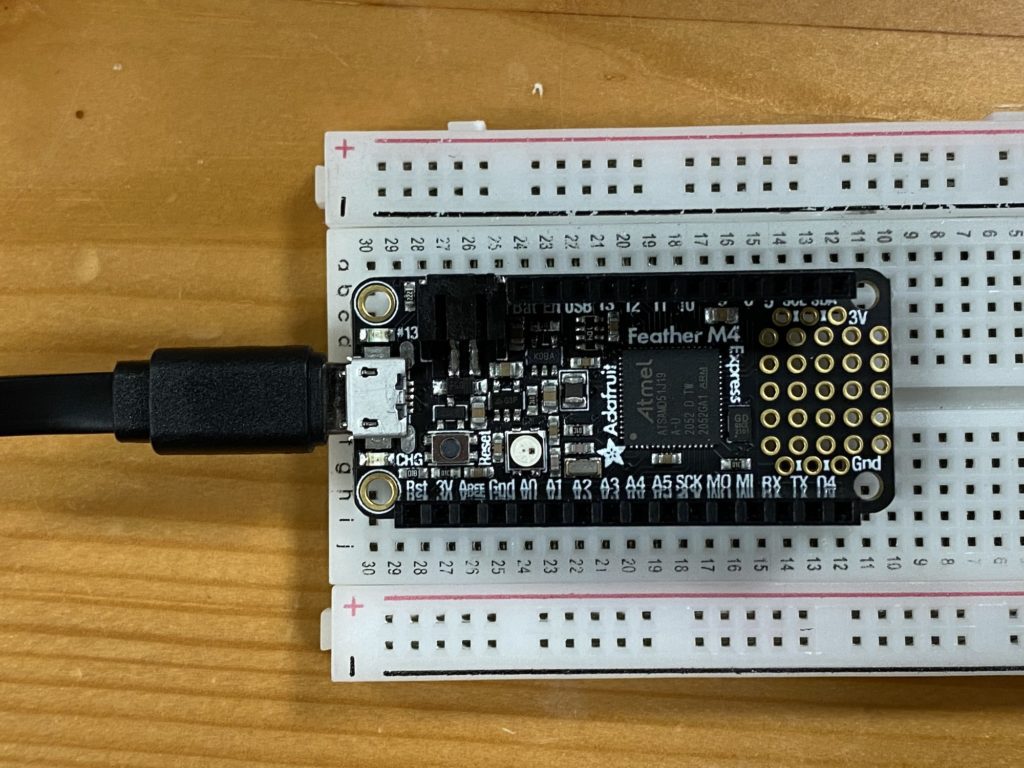
Setup CircuitPython on M4 Express.
Check out the tutorial.
Install the necessary libraries onto your CircuitPython M4 Express:
- Download the CircuitPython libraries 9.x bundle to your computer
- Copy the file lib/adafruit_lis3dh.mpy to your CIRCUITPY/lib folder
Step 2: Make your music
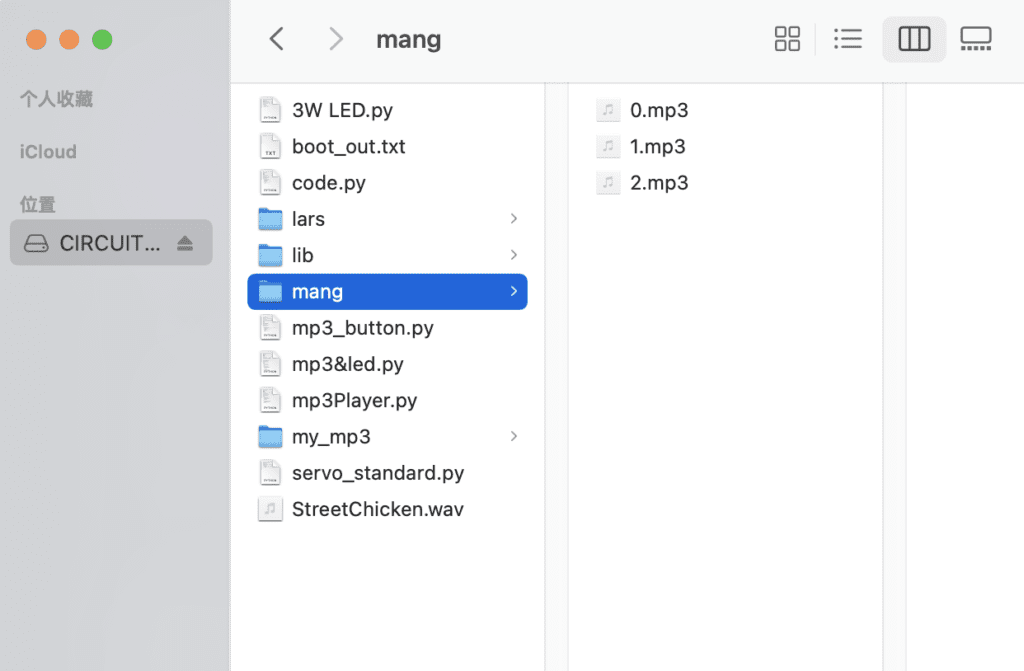
To upload your music onto your board, you can create a folder for your music files.
In this tutorial we create a mang folder inside the CIRCUITPY drive, and we have 3 music files.
Notice: To make your own .mp3, you have to save them with the following settings:
- bit rate: anywhere from 16 to 320 kb/s (lower will be smaller, higher is better quality)
- sample rate: 22050Hz or 44100Hz are recommended, although 16kHz, 24kHz, and 48kHz should also work
- channels: mono or stereo
- must be DRM free
- Recommended settings: 22050Hz, 16kbps, Constant Bit Rate (CB), Mono
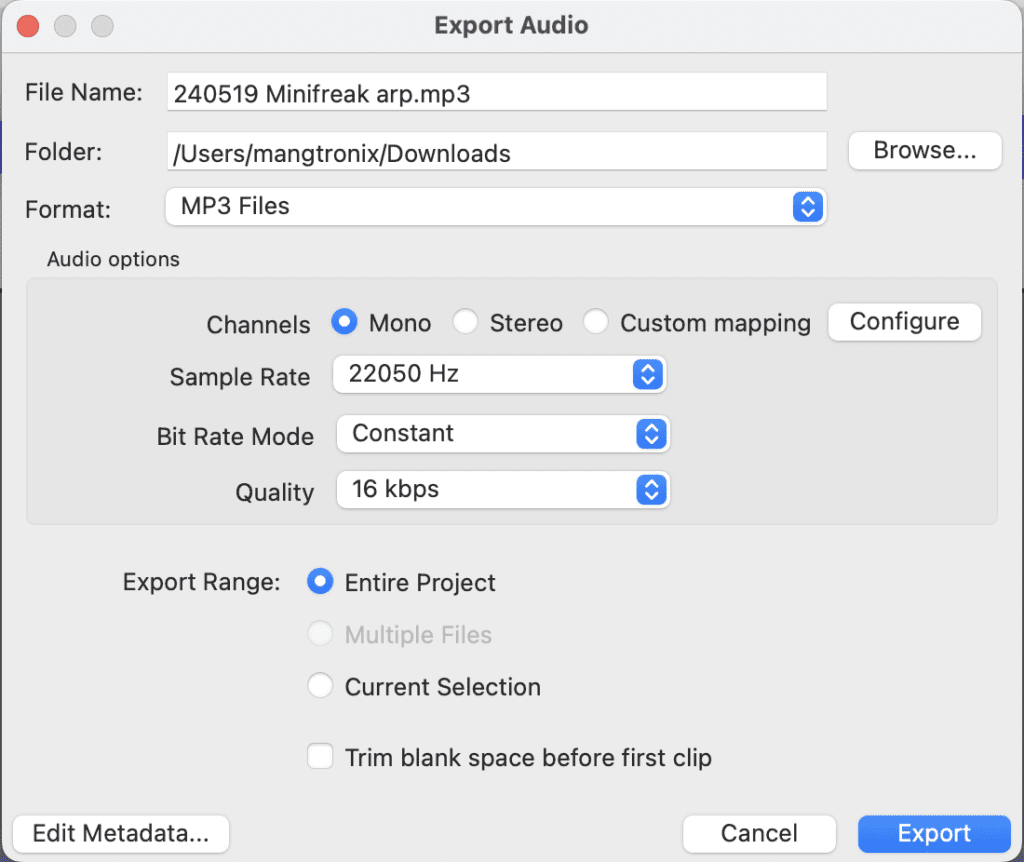
Example export settings with Audacity
Step 3: Build the circuit
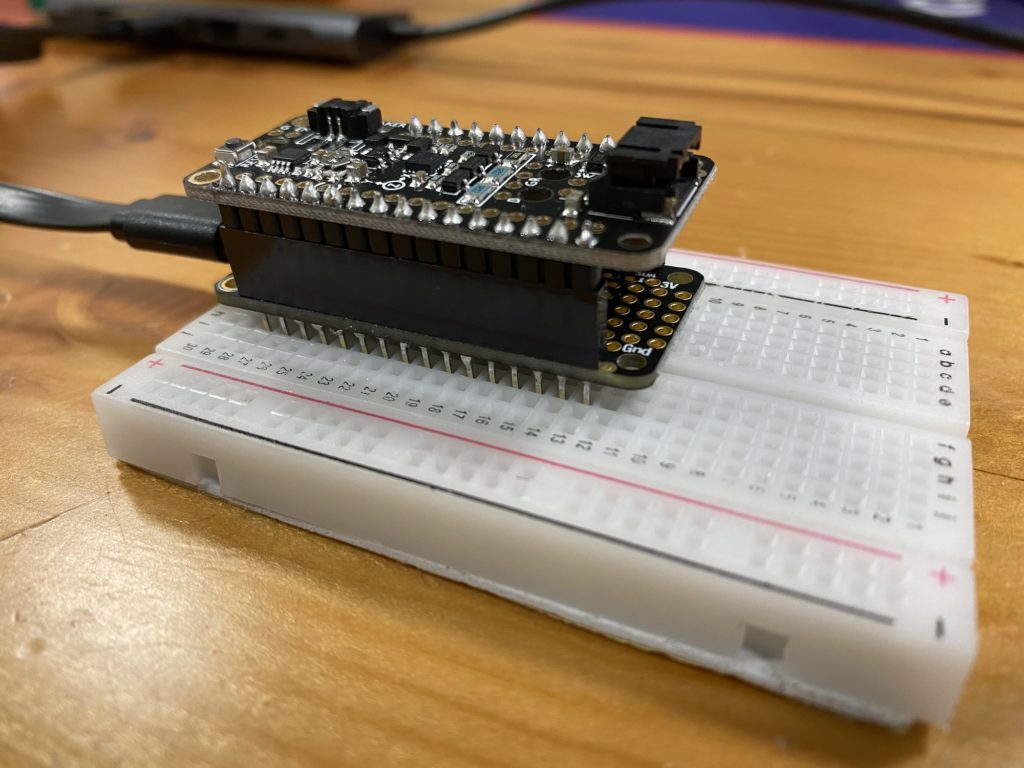
Mark the positions of gound pin (Gnd) and A1 pin.
Stack the Prop-Maker board onto the M4 Express.
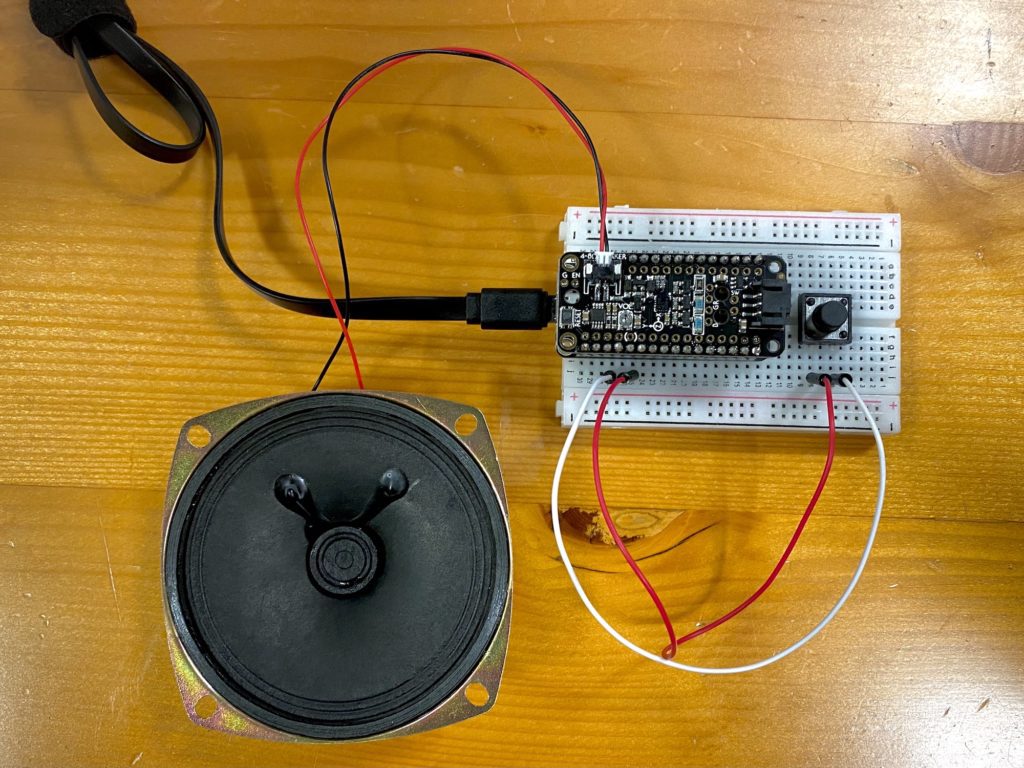
C
Connect the speaker to the Prop-Maker board.
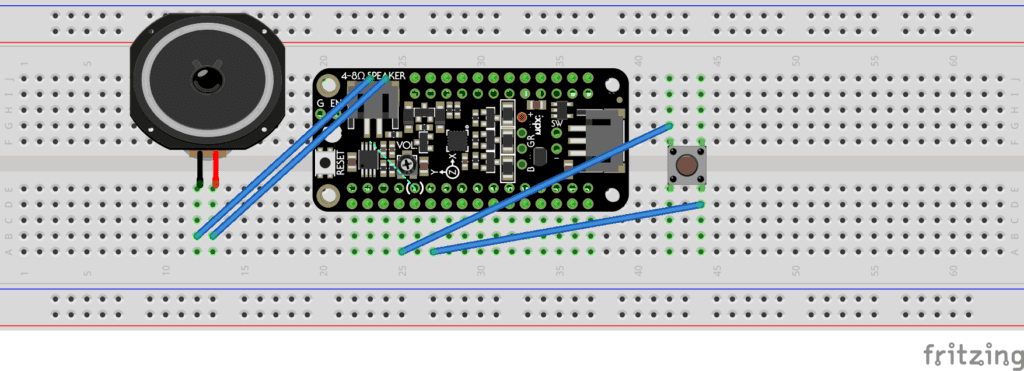
Step 4: Code
Open your code.py file in Mu Editor.
Here is the sample code.
# NYU Abu Dhabi Interactie Media
# Desert Media Art
#
# Tutorial for Sound on M4 Express / Prop-Maker
# Modified by Lydia Yan
#
# SPDX-FileCopyrightText: 2020 Jeff Epler for Adafruit Industries
# SPDX-License-Identifier: MIT
#
# Reference: https://learn.adafruit.com/mp3-circuitpython-lars?view=all#code-dot-py-3064048-3
# https://learn.adafruit.com/circuitpython-essentials/circuitpython-mp3-audio
"""CircuitPython Essentials Audio Out MP3 Example"""
import time
import board
import busio
import digitalio
import audioio
import audiomp3
# Digital input using external push button
button = digitalio.DigitalInOut(board.A1)
button.switch_to_input(pull=digitalio.Pull.UP)
num_sample = 3 # The amount of your mp3 files
sample_number = 0 # Initial played file
# Set up speaker enable pin
enable = digitalio.DigitalInOut(board.D10)
enable.direction = digitalio.Direction.OUTPUT
enable.value = True
# Output from external speaker
speaker = audioio.AudioOut(board.A0)
while True:
# Play your music files in cycle
for i in range(num_sample):
# You may also specify your files' names, here the file names are in number orders starting from 0.
sample = "/mang/{}.mp3".format(sample_number)
mp3stream = audiomp3.MP3Decoder(open(sample, "rb"))
speaker.play(mp3stream)
sample_number = (sample_number + 1) % 10
print("playing", sample)
if i >= (num_sample-1):
sample_number = 0
# This allows you to do other things while the audio plays!
while speaker.playing:
pass
print("Waiting for button press to continue!")
while button.value:
pass
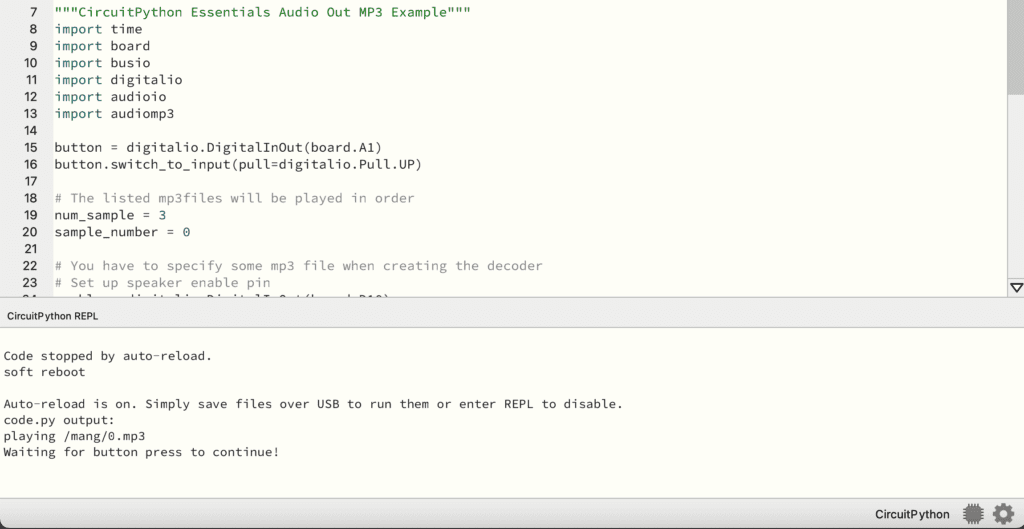
Now the 0.mp3 file is playing through the speaker.
You may also find the status updates in the Serial Console.
If you press the button, you should hear the next music file playing. The music files will loop as a cycle.
0 Comments